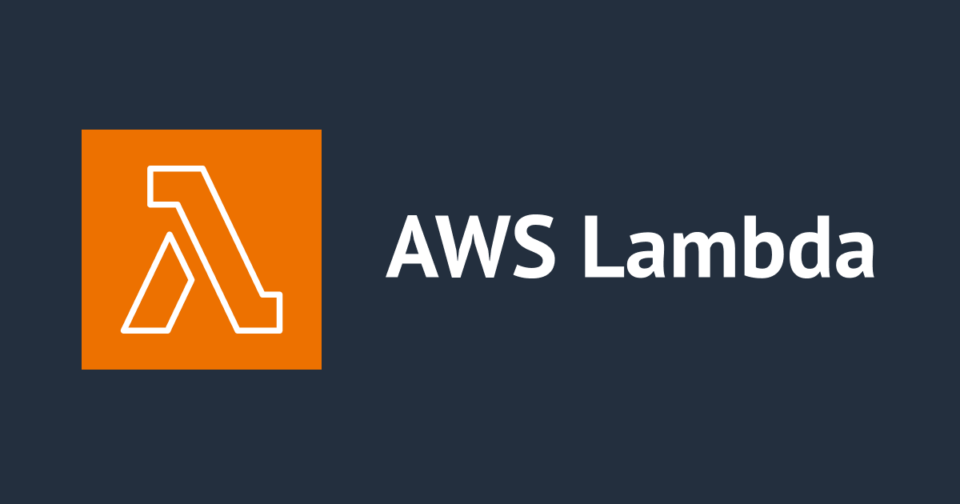
【CDK】API Gateway + Lambdaプロキシ統合にCORS設定を追加する方法
こんにちは、稲葉です。
今回は、AWS CDK(Cloud Development Kit)を使って、API GatewayとLambdaのプロキシ統合にCORS(Cross-Origin Resource Sharing)設定を追加する方法についてご紹介します。
セットアップ
まずは、AWS CDKのプロジェクトをセットアップします。以下のコマンドを使って新しいプロジェクトを作成しましょう。 今回はTypeScriptで実装していきます。
mkdir cdk-proxy-cors cd cdk-proxy-cors cdk init app --language=typescript
構築
lib/cdk-proxy-cors-stack.tsファイルに以下のコードを追加します。
import * as cdk from 'aws-cdk-lib'; import { LambdaIntegration, RestApi } from 'aws-cdk-lib/aws-apigateway'; import { Runtime } from 'aws-cdk-lib/aws-lambda'; import { NodejsFunction } from 'aws-cdk-lib/aws-lambda-nodejs'; import { StringParameter } from 'aws-cdk-lib/aws-ssm'; import { Construct } from 'constructs'; export class CdkProxyCorsStack extends cdk.Stack { constructor(scope: Construct, id: string, props?: cdk.StackProps) { super(scope, id, props); // 今回はCORSのURLをパラメータストアから取得します const cors_value = StringParameter.valueForStringParameter( this, 'CORS_VALUE'); // Lambda Create const goodbyeWorldFunc = new NodejsFunction(this, "goodbyeWorldFunc", { runtime: Runtime.NODEJS_18_X, handler: "handler", entry: 'handlers/goodbye-world-func.ts', environment: { CORS_VALUE: cors_value }, }); // API Gateway Create const restApi = new RestApi(this, "testRestApi", { restApiName: `Rest_API_with_Lambda`, deployOptions: { stageName: 'v1', }, }) const restApiGoodbyeWorld = restApi.root.addResource('goodbye_world'); restApiGoodbyeWorld.addMethod( 'GET', new LambdaIntegration(goodbyeWorldFunc) ); } }
Lambda関数の作成
handlers/goodbye-world-func.tsファイルに関数を実装します。
Lambdaプロキシ統合では、レスポンスがクライアントに直接返されるため、Lambda関数内でヘッダーの付与を行います。(Lambdaプロキシ統合について、詳しく知りたい方は公式ドキュメント:API Gateway で Lambda プロキシ統合を設定するを参照してください)
ヘッダの設定値は公式ドキュメント:REST API リソースの CORS を有効にするを参照しています。
export const handler = async () => { const content = { message: "Goodbye World!" }; const response = { statusCode: 200, headers: { "Content-Type": "application/json", "Access-Control-Allow-Headers" : "Content-Type", "Access-Control-Allow-Origin": `${process.env.CORS_VALUE}`, "Access-Control-Allow-Methods": "OPTIONS,POST,GET" }, body: JSON.stringify(content), }; return response; }
デプロイ
デプロイをする前に、パラメータストアにCORSで設定するURLを設定したいと思います。 AWS Systems Managerを開き、パラメータストアから「パラメータの作成」ボタンを押します。以下の設定で作成します。
- 名前:CORS_VALUE
- 値:https://www.example.com
- 種類:String
- データ型:Text
cdk deploy
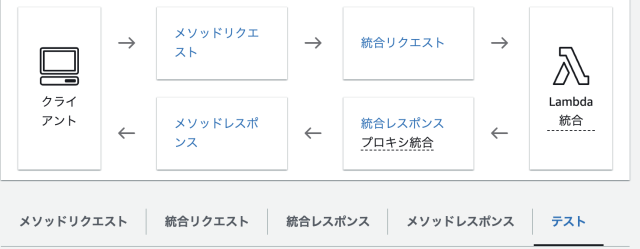
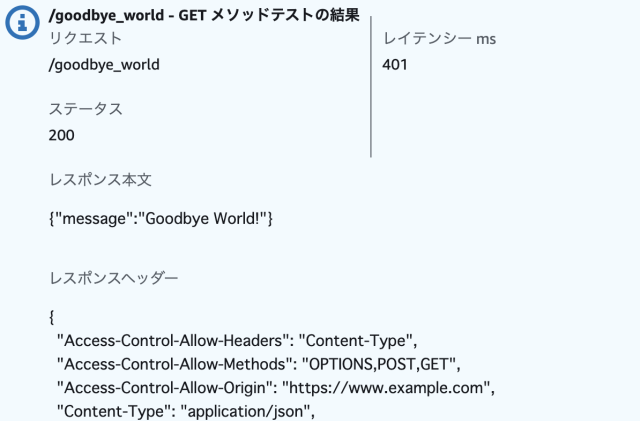
まとめ
AWS CDKを使うことで、API GatewayとLambdaのプロキシ統合にCORS設定を簡単に追加することができました。
最後まで読んでくださりありがとうございました。